目次
オリジナルのボクセルを使って脱出ゲーム制作に挑戦!
用意するもの
- Unity
- VSCode,VisualStudioなど
- 3Dモデル
この記事ではUnity6を使用しています。
アイテムの拡大表示と回転する機能を作成する
前回は、アイテムの選択機能を追加し、それを使ってイベントを発生させる仕組みを作成しました。
今回は取得したアイテムの拡大表示と、マウス操作による回転機能を実装します。
拡大表示でメモを見たり、アイテムの裏側にヒントや暗号を入れ回転機能で見つけるといったことができるようになります。
選択したアイテムを表示するためのUIの作成
まずはアイテムを表示させるためのUIを作成します
UI、背景の作成
新たにCanvasを作成し、その下にPanelを作成
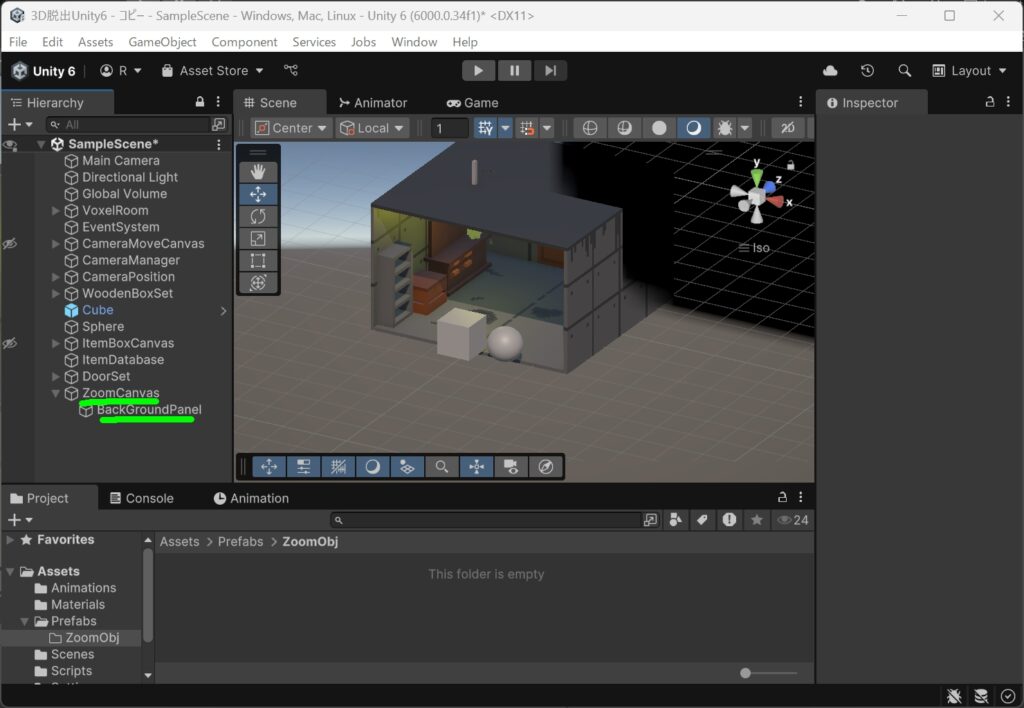
Panelのサイズを変更
画面全体ではなく少し小さめに表示する
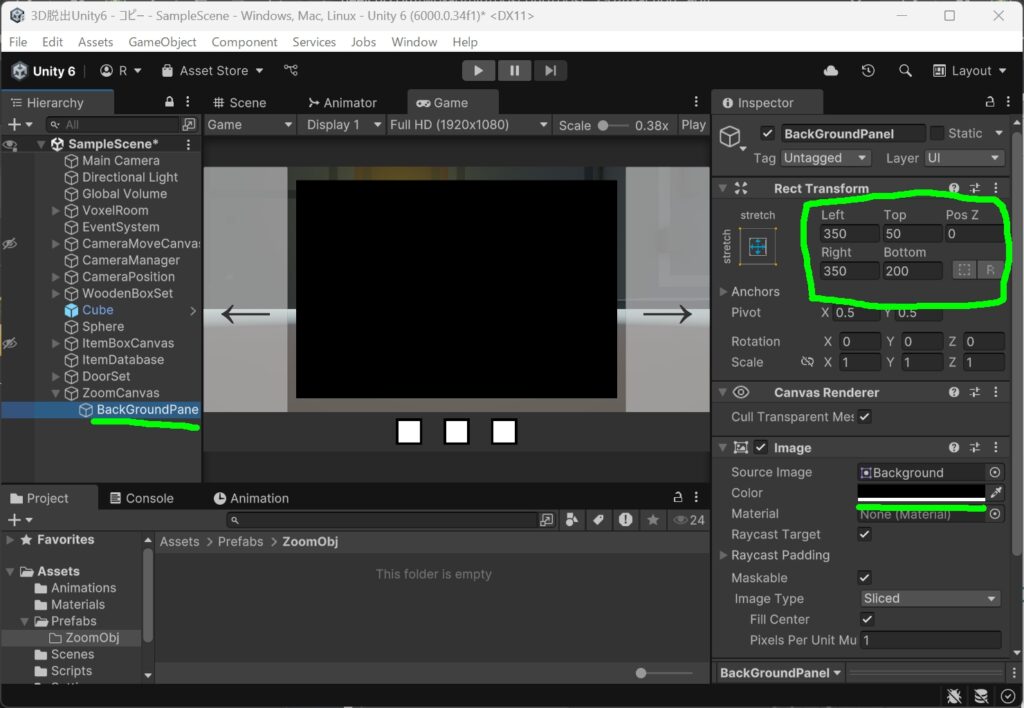
Panelの下にCreateEmptyで『ZoomObjParent』を作成
(このオブジェクトの下にアイテムを配置させます)
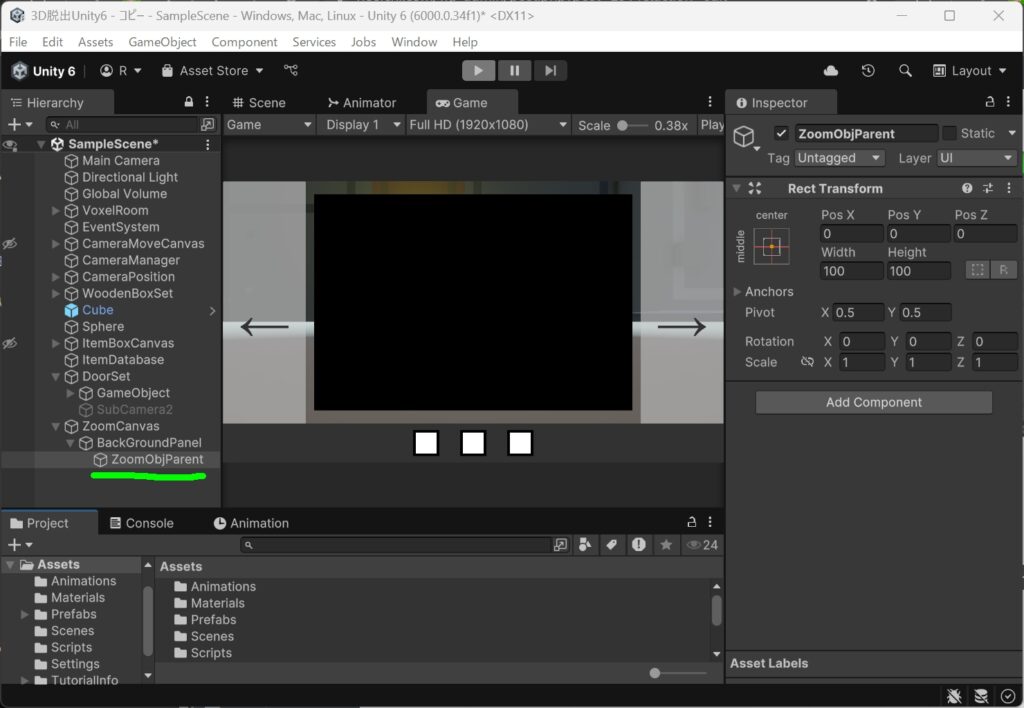
CanvasのRender ModeをScreenSpace-Cameraに変更
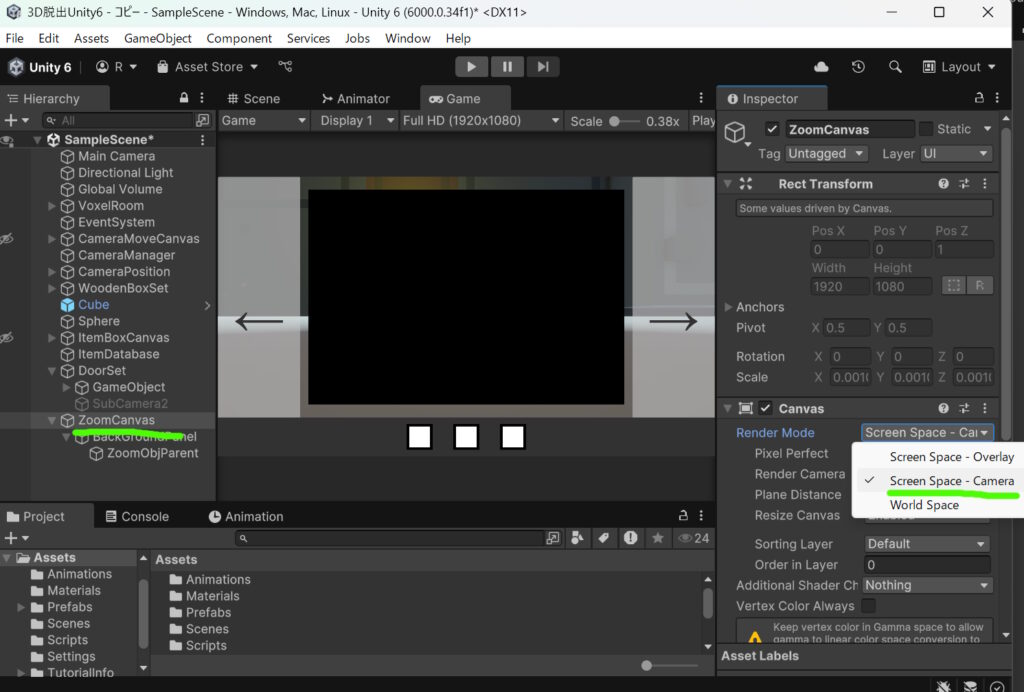
RenderCameraに『MainCamera』をアタッチ
PlaneDistanceを『1』にし、ほかのオブジェクトが手前に表示されないように調整します
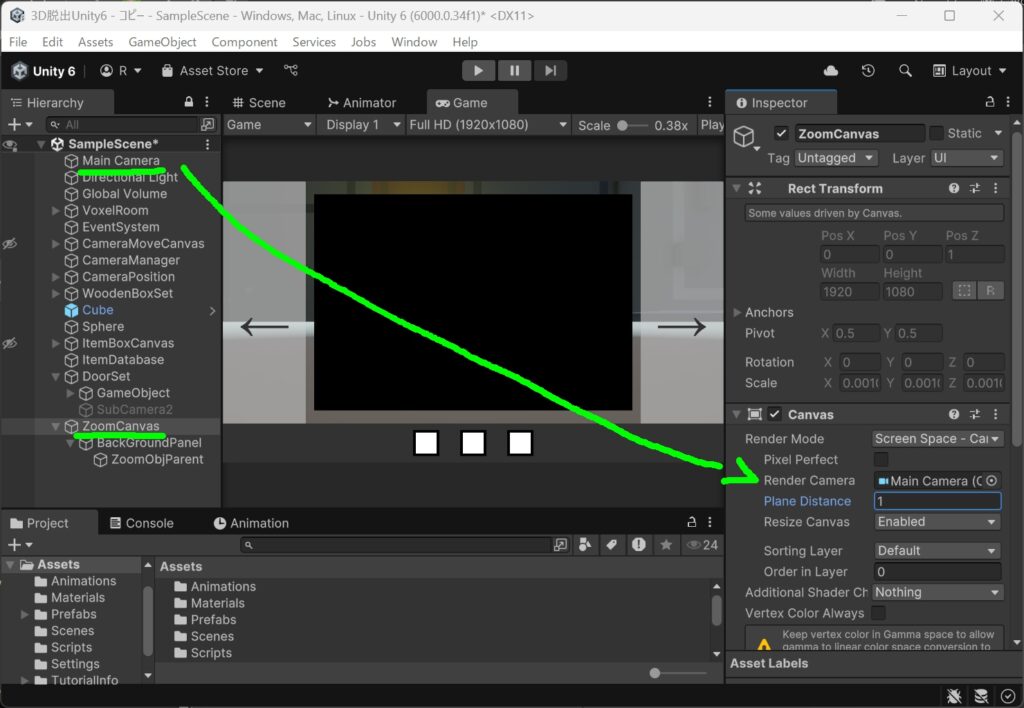
閉じるボタンを作成
パネルを閉じるためのボタンを作成します
Panelの下にimageを作成し位置を調整、AddComponentからButtonを追加しボタン要素にします
UI→Legacy→Textを選択し「×」にしFontsizeを調節
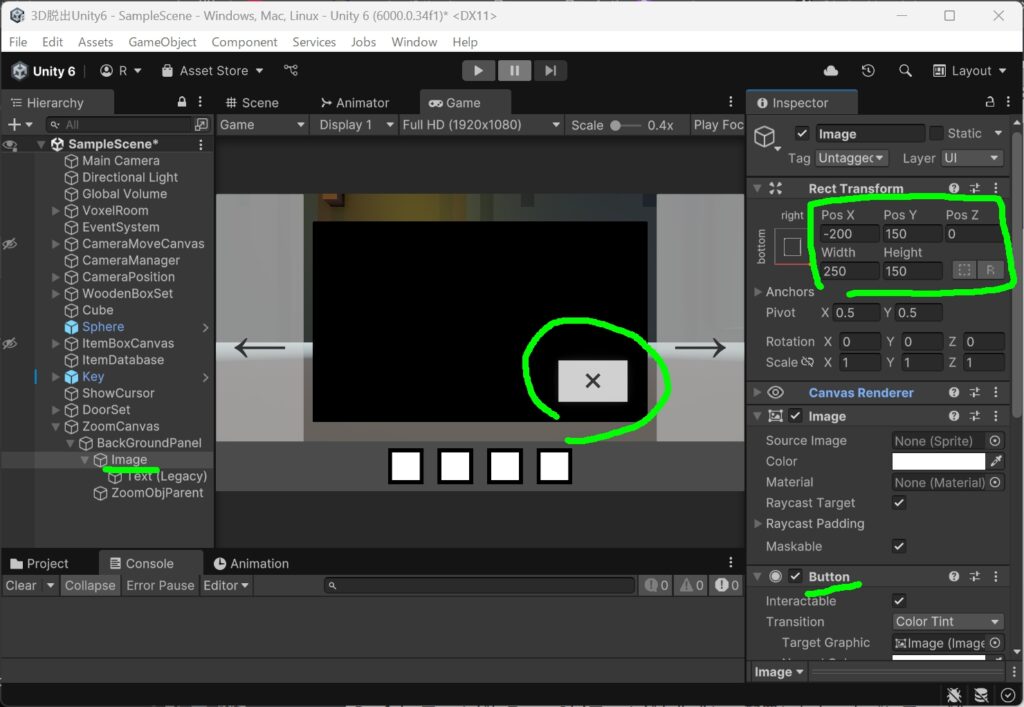
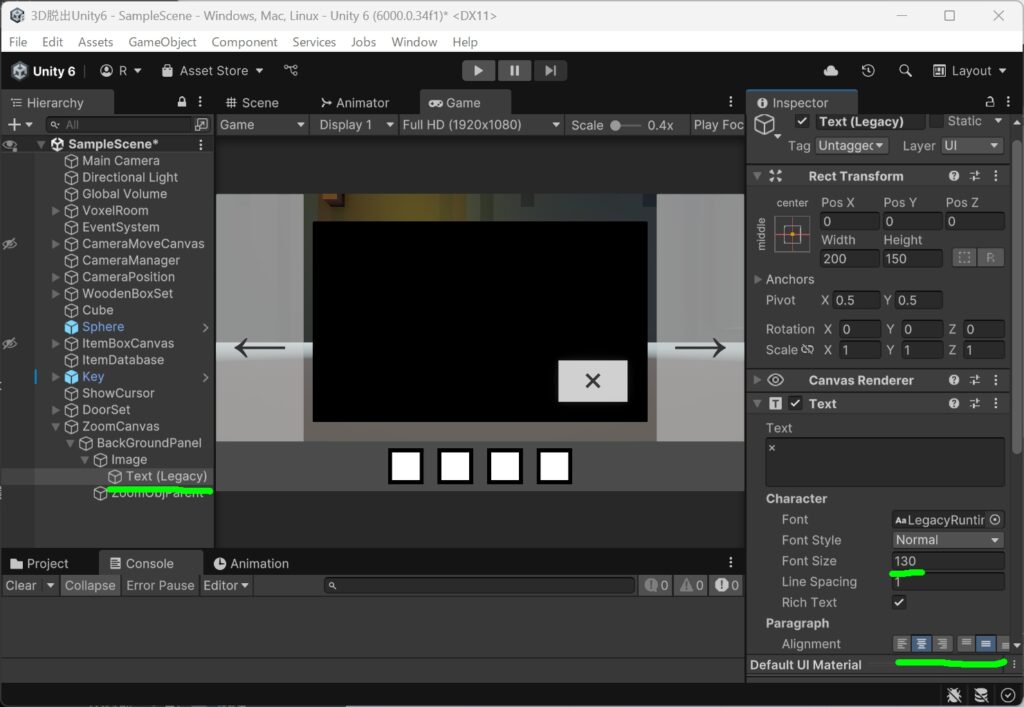
アイテムのプレファブの作成
ZoomObjParentの下にアイテムのモデルをドラッグし追加し、位置や角度を調整
(プレファブ化したものを使用する場合Hierarchyに追加した後UnpackPrefabします)
調整する作業の手間を減らすために、モデルごとに共通する部分はZoomObjParent側で調整します。今回はPositionのZ軸の奥行きと、オブジェクトのスケールを調整。
Rotationでオブジェクトを回転させ、背景のパネルにめりこまないか確認しながら調整します。
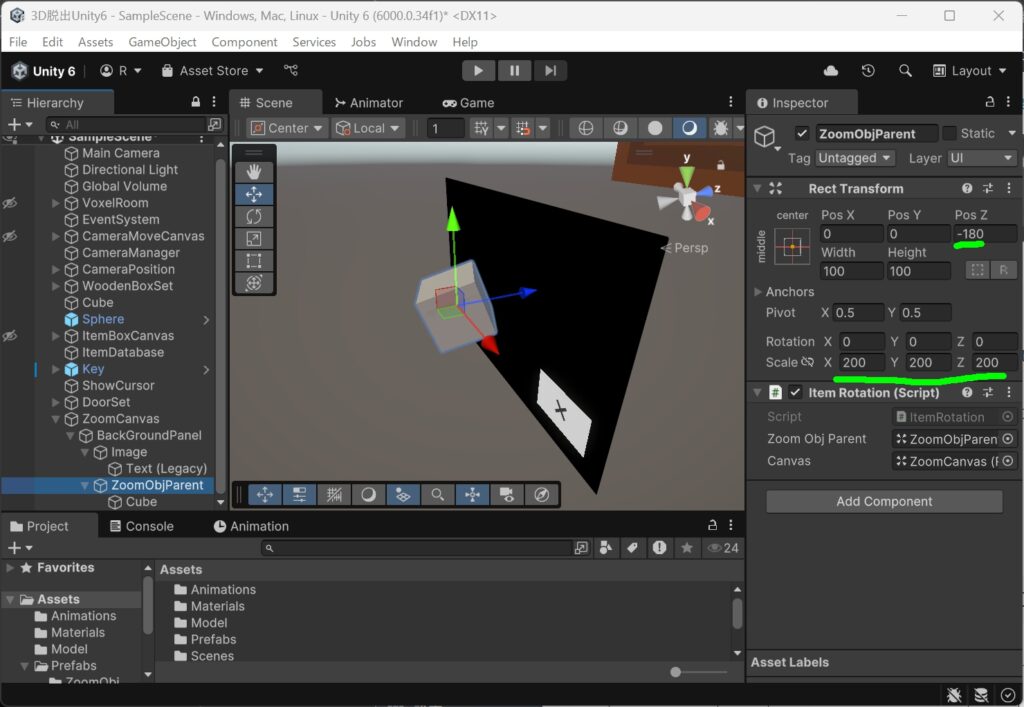
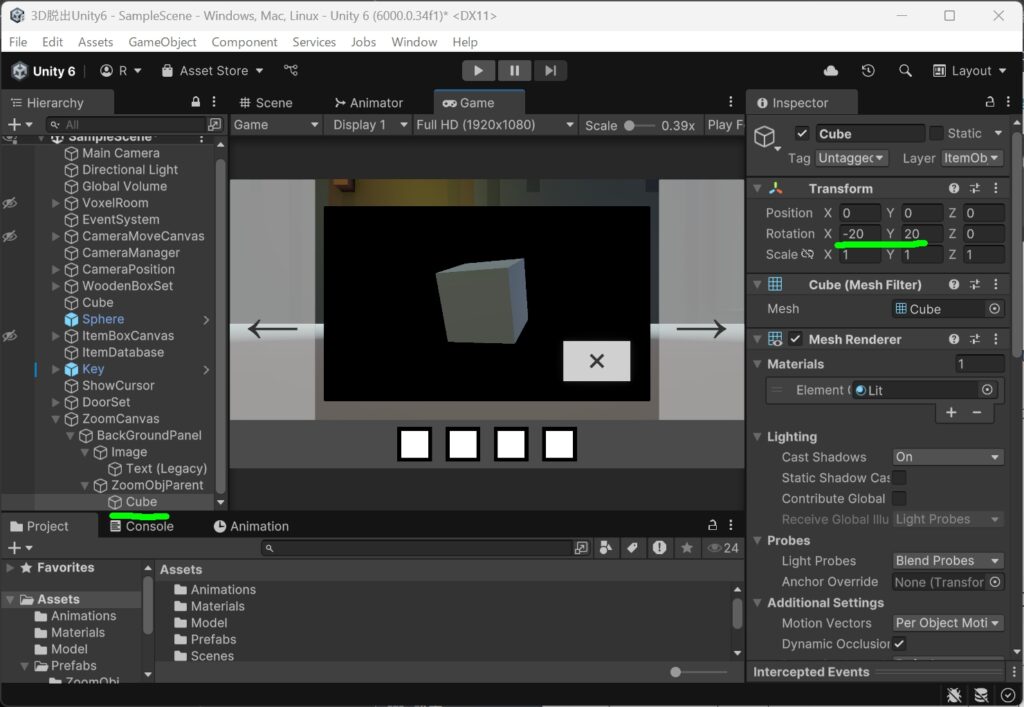
少し傾けてそれっぽく表示したよ
Prefabsフォルダに『ZoomObj』フォルダを作成し、アイテムをプレファブ化
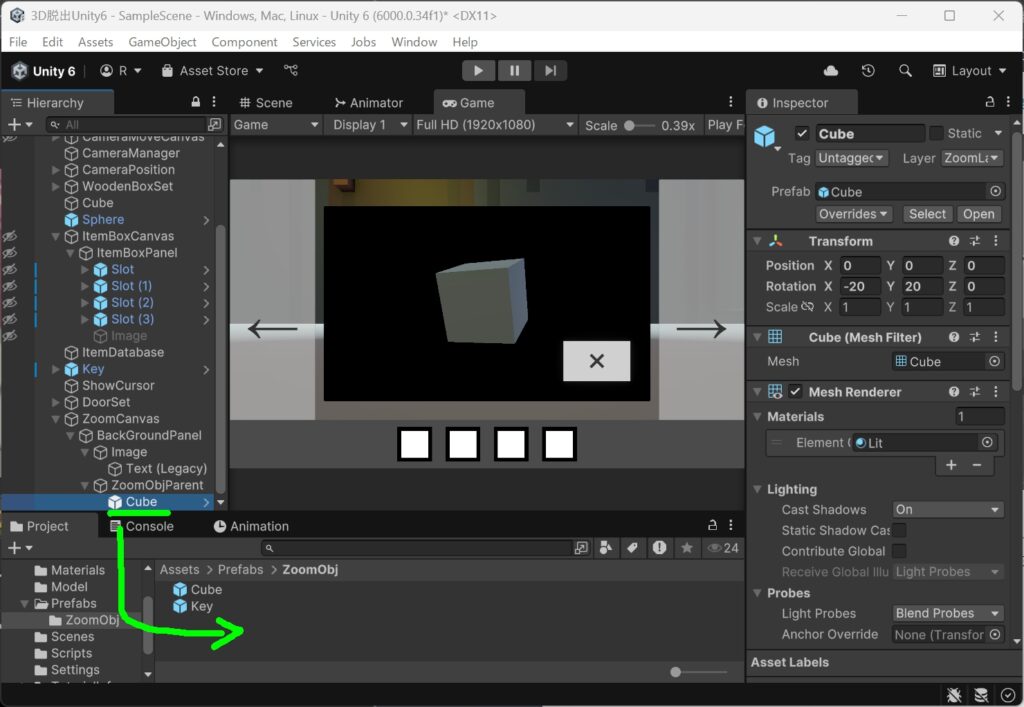
拡大ボタンの作成
アイテムSlotの横に、アイテムを拡大表示するためのボタンを追加
ItemBoxCanvasのItemBoxPanelにimageを追加
AddComponentからButtonを追加しボタン要素にします
このボタンを押したら選択しているアイテムが表示されるようにします。
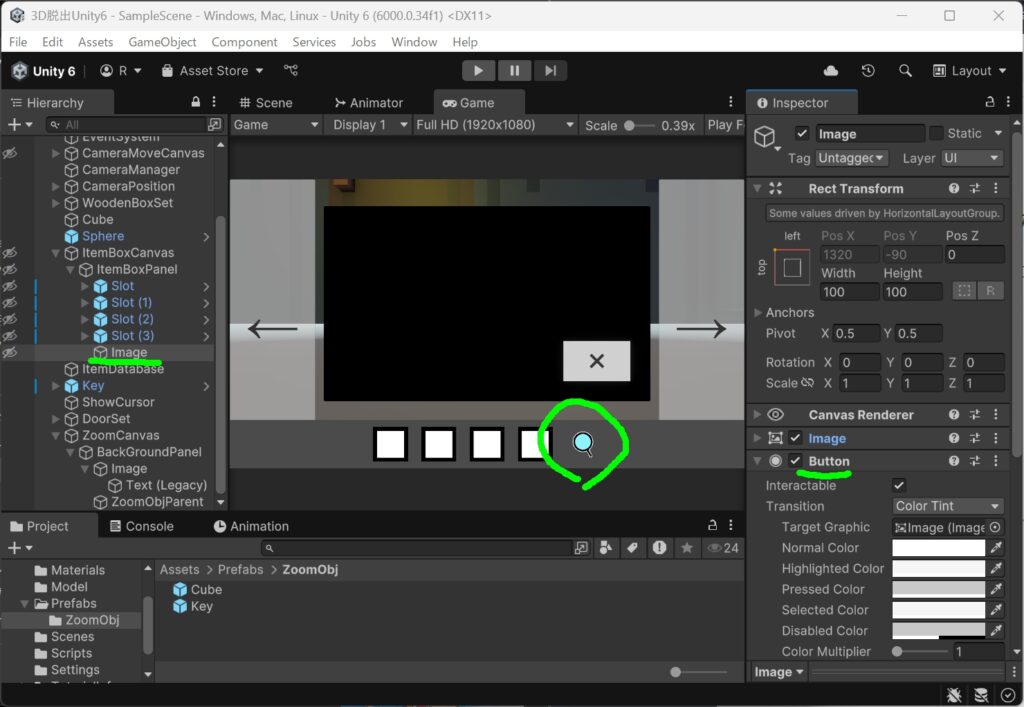
簡単に虫眼鏡の画像を作成したよ
ZoomPanelを表示/非表示する
パネルとアイテムのプレファブが用意できたので、次はスクリプトの作成になります
- UIのパネルを表示/非表示させる
- 選択したアイテムをパネル上に表示させる
- 表示したアイテムをマウス操作で回転できるようにする
スクリプトの作成
まずはUIを表示、非表示するために新しくZoomPanel.csを作成します
ZoomPanel.cs
using UnityEngine;
public class ZoomPanel : MonoBehaviour
{
[SerializeField] GameObject zoomPanel;
void Start()
{
HideZoomPanel();
}
public void OnClickZoom()
{
zoomPanel.SetActive(true);
}
public void HideZoomPanel()
{
zoomPanel.SetActive(false);
}
}
ZoomCanvasにZoomPanel.csをアタッチし、インスペクター上のzoomPanelに対象のPanel (BackGroundPanel)を設定します。
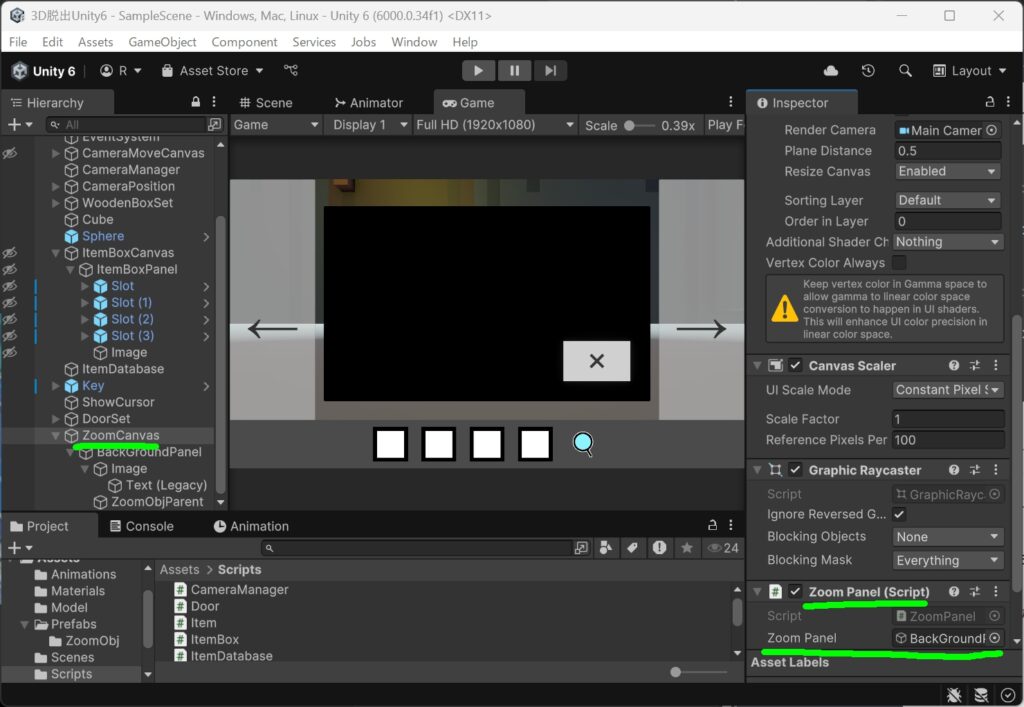
ItemBoxPanelのimage(虫眼鏡)のButtonにOnClickZoomメソッドを設定
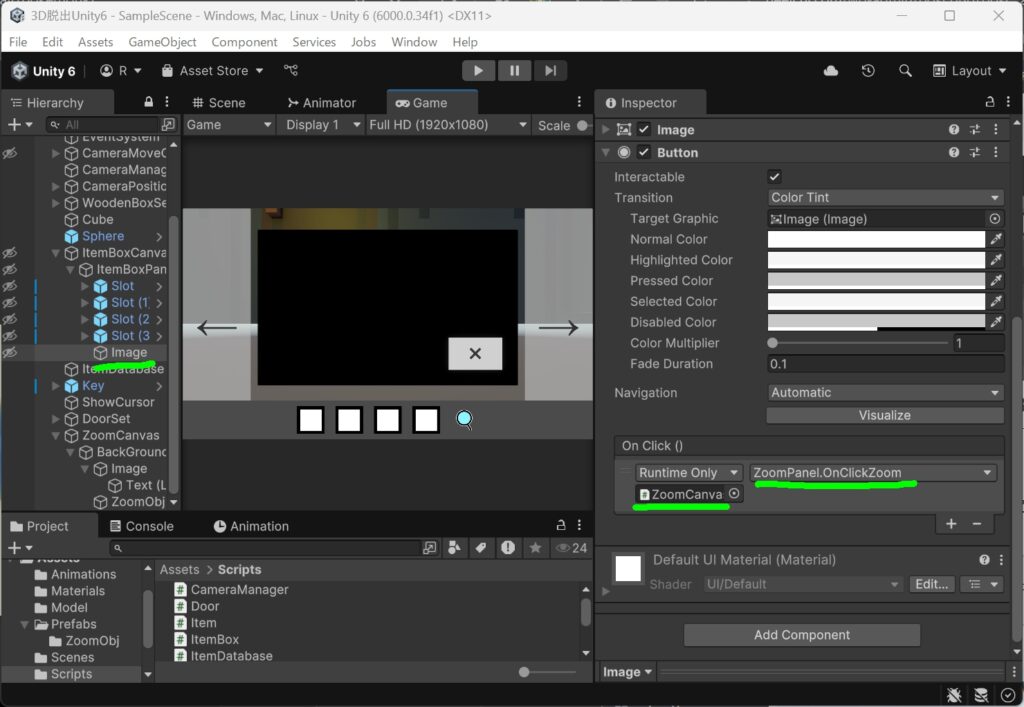
閉じるボタンのButtonにHideZoomPanelメソッドを設定
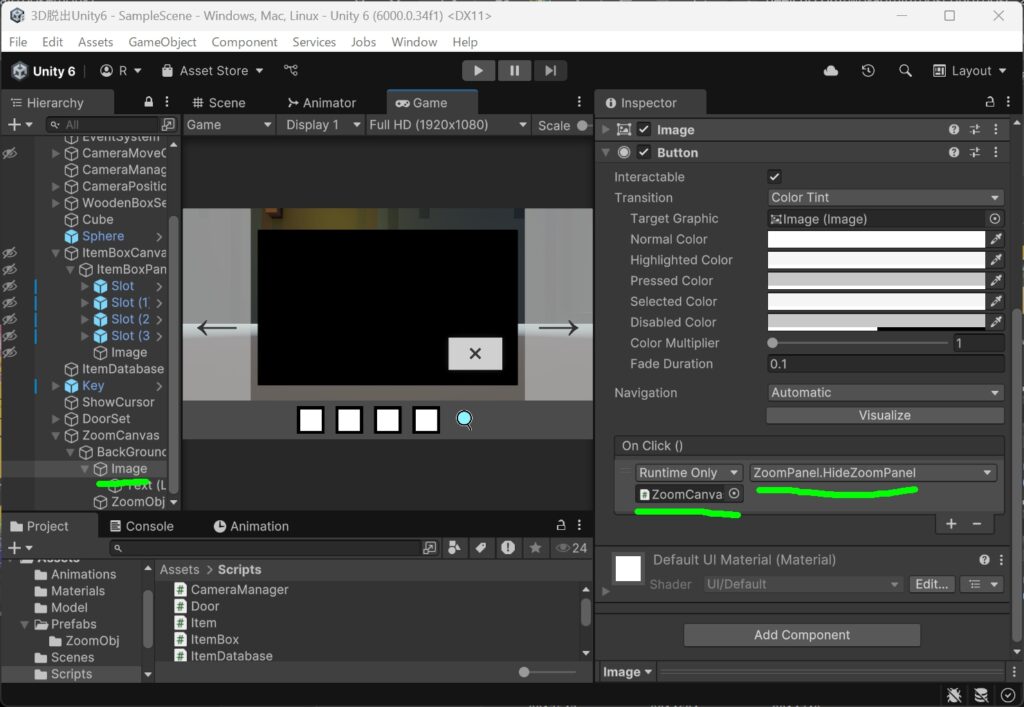
実行
これでパネルの表示非表示ができるようになった
メインカメラ以外でもパネルを出せるようにする
現在ZoomCanvasのRenderCameraがMainCameraになっているので、SubCamera時(木箱などのオブジェクトにズームした時)にはパネルを表示する事ができません
なのでオブジェクトにズームした時にRenderCameraをSubCameraに切り替えるようにします
ZoomPanel.cs
using UnityEngine;
public class ZoomPanel : MonoBehaviour
{
[SerializeField] GameObject zoomPanel;
// 変数を追加
Canvas canvas;
// どこからでもアクセスできるようにする
public static ZoomPanel instance;
private void Awake()
{
instance = this;
}
void Start()
{
// canvasを取得する
canvas = GetComponent<Canvas>();
HideZoomPanel();
}
// 受け取った物をレンダーカメラを入れる
public void SetRenderCamera(Camera camera)
{
canvas.worldCamera = camera;
}
public void OnClickZoom()
{
zoomPanel.SetActive(true);
}
public void HideZoomPanel()
{
zoomPanel.SetActive(false);
}
}
CameraManager.cs
using UnityEngine;
public class CameraManager : MonoBehaviour
{
[SerializeField] GameObject backPanel;
[SerializeField] GameObject movePanels;
public static CameraManager instance;
private void Awake()
{
instance = this;
}
[SerializeField] Transform[] cameraPositions = default;
int currentIndex = 0;
void Start()
{
currentIndex = 0;
mainCamera = Camera.main;
SetCameraPosition(currentIndex);
}
public void TurnRight()
{
currentIndex ++;
if (currentIndex >= cameraPositions.Length)
{
currentIndex = 0;
}
SetCameraPosition(currentIndex);
}
public void TurnLeft()
{
currentIndex--;
if (currentIndex < 0)
{
currentIndex = cameraPositions.Length -1;
}
SetCameraPosition(currentIndex);
}
void SetCameraPosition(int index)
{
mainCamera.transform.position = cameraPositions[index].position;
mainCamera.transform.rotation = cameraPositions[index].rotation;
}
Camera subCamera;
Camera mainCamera;
public void ChangeCamera(Camera subCamera)
{
this.subCamera = subCamera;
backPanel.SetActive(true);
mainCamera.gameObject.SetActive(false);
movePanels.SetActive(false);
// レンダーカメラをSubCameraに設定
ZoomPanel.instance.SetRenderCamera(subCamera);
}
public void OnBackButton()
{
backPanel.SetActive(false);
this.subCamera.gameObject.SetActive(false);
mainCamera.gameObject.SetActive(true);
movePanels.SetActive(true);
// レンダーカメラをMainCameraに設定
ZoomPanel.instance.SetRenderCamera(mainCamera);
}
}
ChangeCameraメソッドとOnBackButtonメソッドにSetRenderCameraを実行するものを追加
これでカメラが切り替わった際に、ZoomCanvasのレンダーカメラも切り替わるようになりました。
選択したアイテムをZoomPanelに表示する
次は選択したアイテムの情報を受け取り、データベースを参照しプレファブを表示させます
データベースに表示するプレファブを追加
データベースに表示するプレファブの項目を増やします
Item.cs
using UnityEngine;
using System;
[Serializable]
public class Item
{
public enum Type
{
Cube,
Sphere,
Key,
}
public Type type;
public Sprite sprite;
// Zoom時に表示するためのPrefab
public GameObject zoomPrefab;
public Item(Item item)
{
this.type = item.type;
this.sprite = item.sprite;
}
}
ZoomObjフォルダのプレファブをデータベースに設定
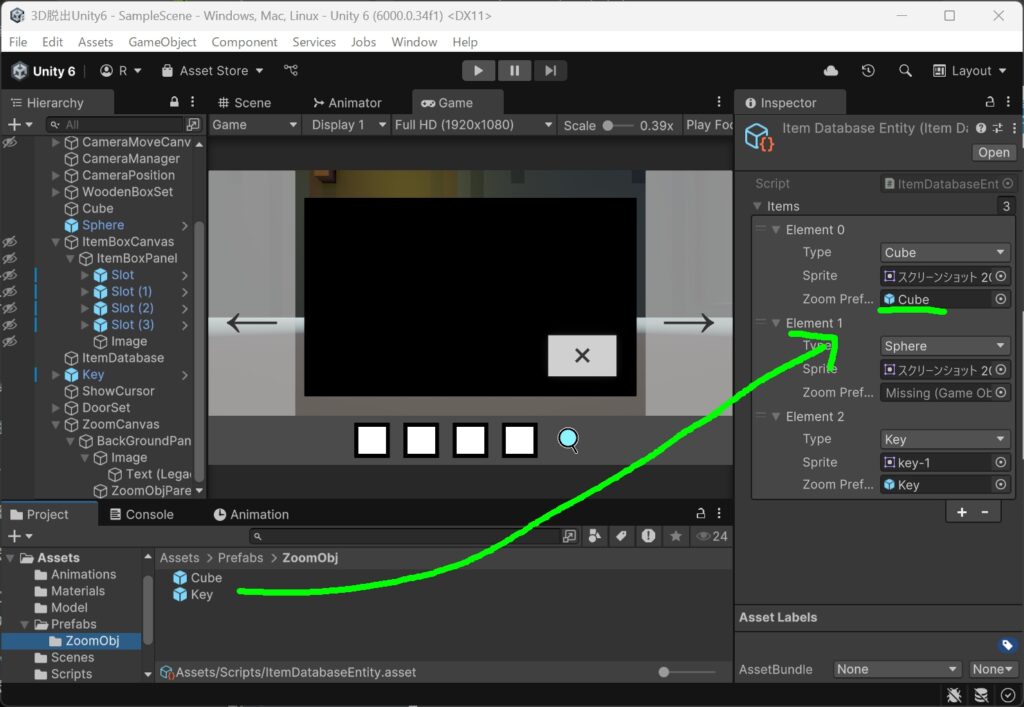
ItemDatabase.cs
using System.Threading;
using UnityEngine;
public class ItemDatabase : MonoBehaviour
{
public static ItemDatabase instance;
private void Awake()
{
instance = this;
}
[SerializeField] ItemDatabaseEntity ItemDatabaseEntity;
public Item Spawn(Item.Type type)
{
for (int i = 0; i < ItemDatabaseEntity.items.Count; i++)
{
Item itemData = ItemDatabaseEntity.items[i];
if (itemData.type == type)
{
return new Item(itemData);
}
}
return null;
}
// ズームアイテムを生成する
public GameObject CreateZoomItem(Item.Type itemType)
{
for (int i = 0; i < ItemDatabaseEntity.items.Count; i++)
{
Item itemData = ItemDatabaseEntity.items[i];
if (itemData.type == itemType)
{
return Instantiate(itemData.zoomPrefab);
}
}
return null;
}
}
ItemDatabase.csにプレファブを生成するCreateZoomItemメソッドを追加します
ItemBox.cs
using UnityEngine;
public class ItemBox : MonoBehaviour
{
[SerializeField] Slot[] slots;
public static ItemBox instance;
Slot selectSlot;
private void Awake()
{
instance = this;
}
public void SetItem(Item item)
{
for (int i = 0; i < slots.Length; i++)
{
Slot slot = slots[i];
if (slot.IsEmpty())
{
slot.Set(item);
break;
}
}
}
public void OnSlotClick(int position)
{
for (int i = 0; i < slots.Length; i++)
{
slots[i].HideBackPanel();
}
slots[position].OnSelect();
selectSlot = slots[position];
}
public bool CheckSelectItem(Item.Type useItemType)
{
if (selectSlot == null)
{
return false;
}
if (selectSlot.GetItem().type == useItemType)
{
return true;
}
return false;
}
public void UseSelectItem()
{
selectSlot.RemoveItem();
selectSlot = null;
}
// 選択したスロットを取得する
public Item GetSelectItem()
{
if (selectSlot == null)
{
return null;
}
return selectSlot.GetItem();
}
}
ItemBox.csにGetSelectItemメソッドの追加
ZoomPanel.cs
using UnityEngine;
public class ZoomPanel : MonoBehaviour
{
[SerializeField] GameObject zoomPanel;
Canvas canvas;
// 生成する親を設定
[SerializeField] Transform zoomObjParent;
// zoomItemの変数を作成
GameObject zoomItem;
public static ZoomPanel instance;
private void Awake()
{
instance = this;
}
void Start()
{
canvas = GetComponent<Canvas>();
HideZoomPanel();
}
public void SetRenderCamera(Camera camera)
{
canvas.worldCamera = camera;
}
public void OnClickZoom()
{
// アイテムを選択されていなければ実行しない
Item selectItem = ItemBox.instance.GetSelectItem();
if (selectItem == null)
{
return;
}
zoomPanel.SetActive(true);
// 追加
ShowItem();
}
// 選択したアイテムを表示する
void ShowItem()
{
// 既にzoomItemがあるなら重複させないために削除する
if (zoomItem != null)
{
Destroy(zoomItem);
}
// 選択したアイテムの情報を取得
Item selectItem = ItemBox.instance.GetSelectItem();
// プレファブを生成する
GameObject zoomItem = ItemDatabase.instance.CreateZoomItem(selectItem.type);
// 生成する場所を指定
zoomItem.transform.SetParent(zoomObjParent);
}
public void HideZoomPanel()
{
zoomPanel.SetActive(false);
}
}
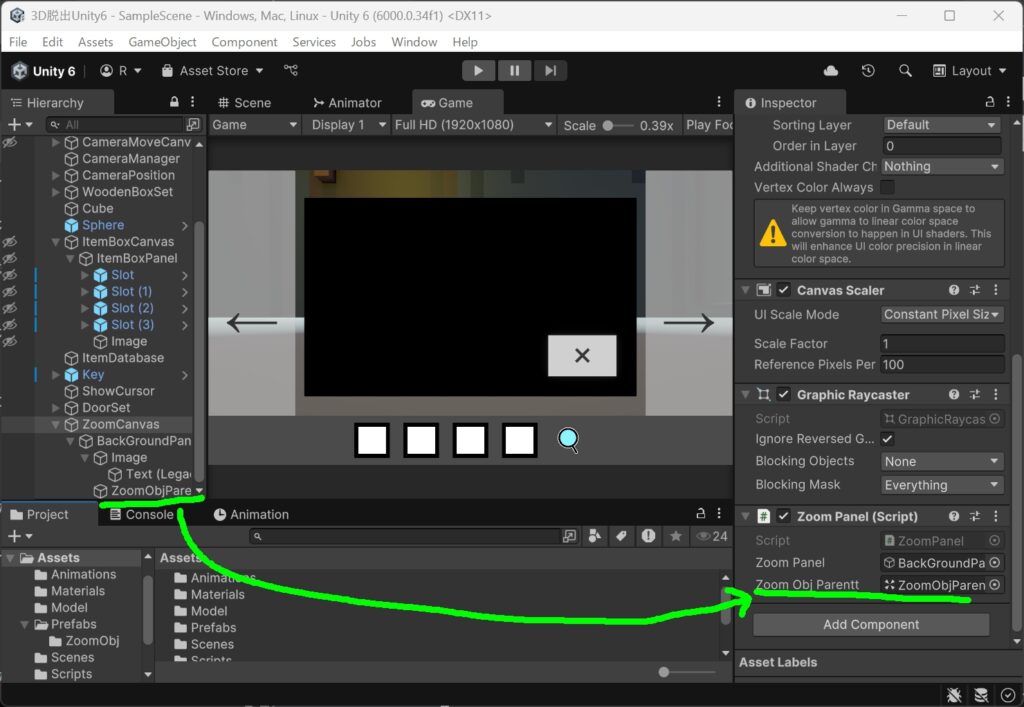
インスペクター上のzoomObjParentに対象のものを設定します。
実行
マウス操作で表示しているアイテムを回転させる
次はマウスをドラッグして表示したアイテムを回転させる仕組みを作成します
ItemRotation.cs
using UnityEngine;
public class ItemRotation : MonoBehaviour
{
// ズーム表示時のアイテムの親オブジェクト
[SerializeField] Transform zoomObjParent;
// キャンバス(回転軸の基準にする)
[SerializeField] Transform canvas;
// 回転速度
float speed = 20;
void Update()
{
// 左クリックを押している間、アイテムを回転させる
if (Input.GetMouseButton(0))
{
// マウスの横移動で左右回転(X軸回転)
float dx = -Input.GetAxis("Mouse X") * speed;
// マウスの縦移動で上下回転(Y軸回転)
float dy = Input.GetAxis("Mouse Y") * speed;
// アイテムをキャンバスの回転を基準に回転させる
zoomObjParent.RotateAround(transform.position, canvas.transform.rotation * Vector3.up, dx);
zoomObjParent.RotateAround(transform.position, canvas.transform.rotation * Vector3.right, dy);
}
}
}
インスペクター上のzoomObjParentとcanvasに対象のものを設定します。
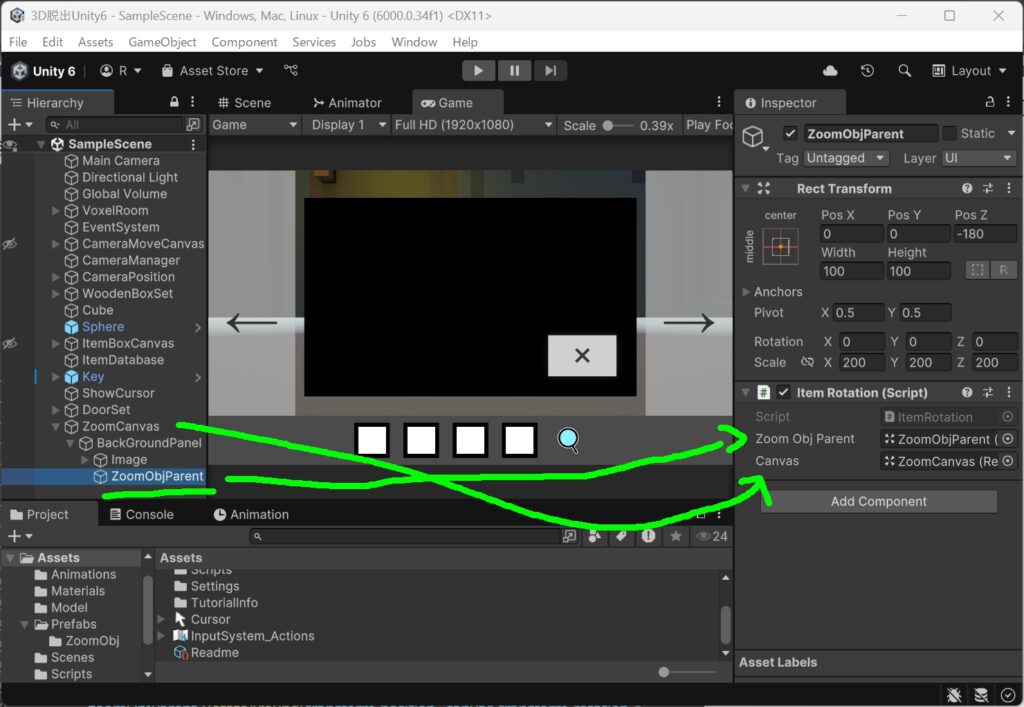
実行
ドラッグして回転させられるようになった
ZoomObjParentのRotationをリセットする
現在、アイテムを回転させた後もZoomObjParentのRotationが維持されるため、別のアイテムを表示した際に意図しない角度で表示されてしまいます。
そこで、アイテムを表示するたびにRotationをリセットする処理を追加し、常に正しい向きで表示されるようにします。
ResetRotation.cs
using UnityEngine;
public class ItemRotation : MonoBehaviour
{
[SerializeField] Transform zoomObjParent;
[SerializeField] Transform canvas;
float speed = 20;
// シングルトン化を追加
public static ItemRotation instance;
private void Awake()
{
if (instance == null)
{
instance = this;
}
else
{
Destroy(gameObject);
}
}
void Update()
{
if (Input.GetMouseButton(0))
{
float dx = -Input.GetAxis("Mouse X") * speed;
float dy = Input.GetAxis("Mouse Y") * speed;
zoomObjParent.RotateAround(transform.position, canvas.transform.rotation*Vector3.up, dx);
zoomObjParent.RotateAround(transform.position, canvas.transform.rotation*Vector3.right, dy);
}
}
// 回転をリセットするメソッドを追加
public void ResetRotation()
{
zoomObjParent.localRotation = Quaternion.identity;
}
}
ResetRotationメソッドを追加し、スクリプトをシングルトン化
ZoomPanel.cs
using UnityEngine;
public class ZoomPanel : MonoBehaviour
{
[SerializeField] GameObject zoomPanel;
Canvas canvas;
[SerializeField] Transform zoomObjParentt;
GameObject zoomItem;
public static ZoomPanel instance;
private void Awake()
{
instance = this;
}
void Start()
{
canvas = GetComponent<Canvas>();
HideZoomPanel();
}
public void SetRenderCamera(Camera camera)
{
canvas.worldCamera = camera;
}
public void OnClickZoom()
{
Item selectItem = ItemBox.instance.GetSelectItem();
if (selectItem == null)
{
return;
}
zoomPanel.SetActive(true);
ShowItem();
}
//
void ShowItem()
{
if (zoomItem != null)
{
Destroy(zoomItem);
}
Item selectItem = ItemBox.instance.GetSelectItem();
zoomItem = ItemDatabase.instance.CreateZoomItem(selectItem.type);
zoomItem.transform.SetParent(zoomObjParentt, false);
// シングルトン経由で ResetRotation を呼び出し
ItemRotation.instance.ResetRotation();
}
public void HideZoomPanel()
{
zoomPanel.SetActive(false);
}
}
ShowItemメソッドでResetRotationを実行
実行
いい感じになった
不具合の修正
いくつか細かい不具合があるので修正します
拡大表示中は視点移動をできないようにする
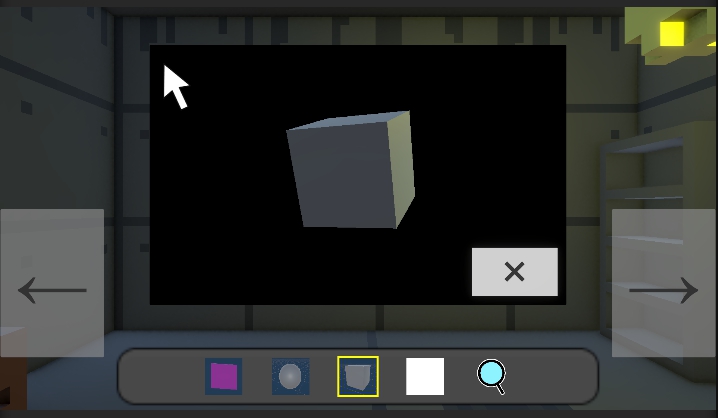
アイテムを拡大表示しているとき、他のUIが残っていています。
このままでは拡大したまま視点移動ができてしまうので修正します。
スクリプトの修正
SerializeFieldで視点移動するUIの元になっているCanvasを取得します。
ZoomPanel.csにCanvasの表示を変えるSetActiveを追加します。
ZoomPanel.cs
// CameraMoveCanvasを取得
[SerializeField] GameObject moveCanvas;
public void OnClickZoom()
{
Item selectItem = ItemBox.instance.GetSelectItem();
if (selectItem == null)
{
return;
}
zoomPanel.SetActive(true);
ShowClickItem()
// Canvasを非表示にする
moveCanvas.SetActive(false);
}
public void HideZoomPanel()
{
zoomPanel.SetActive(false);
// Canvasを表示する
moveCanvas.SetActive(true);
}
InspectorのmoveCanvasにCameraMoveCanvasを設定
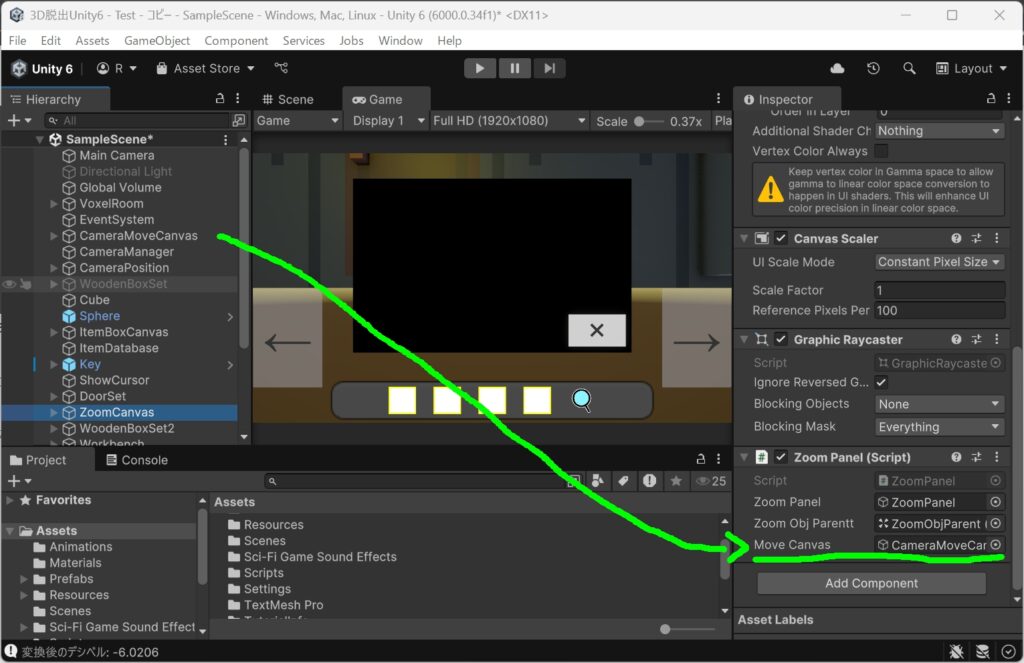
これで拡大表示中に視点移動ができなくなった
拡大表示中はオブジェクトをクリックできないようにする
拡大表示中にオブジェクトをクリックできてしまうのでこちらも修正します。
全体を覆うパネルを追加しオブジェクトをクリックできないようにします。
新しくパネルを作成
ZoomCanvasを右クリックしPanelを追加し、名前を「ZoomPanel」にします。
色を透明にし、RayCastTargetにチェックが入ってるのを確認。
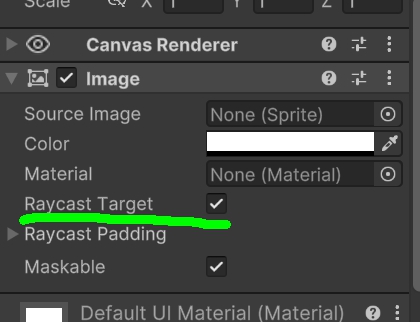
BackGroundPanel、image、ZoomObjParentをZoomPanelの子要素にします。
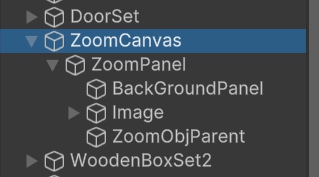
スクリプトのボタンによる表示非表示の対象をBackGroundPanelからZoomPanelに変更します。
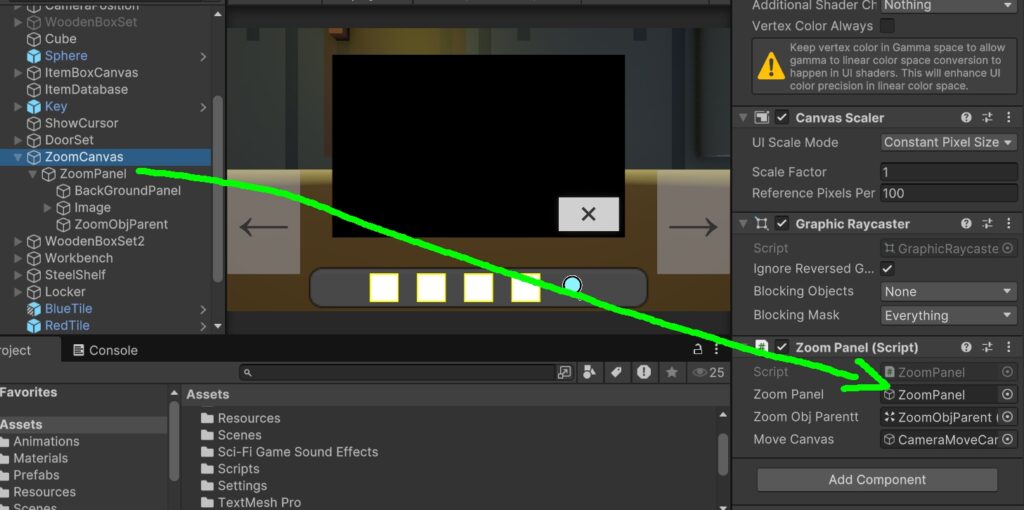
実行
OK!
まとめ
今回の実装では、取得したアイテムの拡大表示と、マウス操作による回転機能を追加しました。これにより、アイテムの細かい部分を確認したり、裏側に隠されたヒントを見つけたりすることができるようになりました。
この仕組みを活用すれば、鍵やスイッチの詳細を調べたり、アイテムを特定の角度で組み合わせるなど、より奥深い謎解きを作ることができます。
とりあえず今回はここまで